
Batch Apex in Salesforce Tutorial
Last updated on 08th Oct 2020, Blog, Tutorials
What is Batch Apex in Salesforce?
Batch class in salesforce is used to run large jobs (think thousands or millions of records!) that would exceed normal processing limits. Using Batch Apex, you can process records asynchronously in batches (hence the name, “Batch Apex”) to stay within platform limits. If you have a lot of records to process, for example, data cleansing or archiving, Batch Apex is probably your best solution.
Here’s how Batch Apex works under the hood. Let’s say you want to process 1 million records using Batch Apex. The execution logic of the batch class is called once for each batch of records you are processing. Each time you invoke a batch class, the job is placed on the Apex job queue and is executed as a discrete transaction.
Advantage of using batch Apex
- Every transaction starts with a new set of governor limits, making it easier to ensure that your code stays within the governor execution limits.
- If one batch fails to process successfully, all other successful batch transactions aren’t rolled back
Batch Apex Syntax
To write a Batch Apex class, your class must implement the Database.Batchable interface and include the following three methods:
Start
Start method is automatically called at the beginning of the apex job. This method will collect records or objects on which the operation should be performed. These records are divided into subtasks and pass those to the execute method.
Used to collect the records or objects to be passed to the interface method executed for processing. This method is called once at the beginning of a Batch Apex job and returns either a Database.QueryLocator object or an Iterable that contains the records or objects passed to the job.
Most of the time a QueryLocator does the trick with a simple SOQL query to generate the scope of objects in the batch job. But if you need to do something crazy like loop through the results of an API call or pre-process records before being passed to the execute method, you might want to check out the Custom Iterators link in the Resources section.
With the QueryLocator object, the governor limit for the total number of records retrieved by SOQL queries is bypassed and you can query up to 50 million records. However, with an Iterable, the governor limit for the total number of records retrieved by SOQL queries is still enforced.
Subscribe For Free Demo
Error: Contact form not found.
Execute
Execute Method performs an operation which we want to perform on the records fetched from start method.
Performs the actual processing for each chunk or “batch” of data passed to the method. The default batch size is 200 records. Batches of records are not guaranteed to execute in the order they are received from the start method.
This method takes the following:
- A reference to the Database.BatchableContext object.
- A list of sObjects, such as List<sObject>, or a list of parameterized types. If you are using a Database.QueryLocator, use the returned list.
Finish
Finish method executes after all batches are processed. Use this method to send confirmation email notifications
Used to execute post-processing operations (for example, sending an email) and is called once after all batches are processed.
Here’s what the skeleton of a Batch Apex class looks like:
- global class MyBatchClass implements Database.Batchable<sObject>
- global (Database.QueryLocator | Iterable<sObject>) start(Database.BatchableContext bc) {
- // collect the batches of records or objects to be passed to execute
- global void execute(Database.BatchableContext bc, List<P> records){
- // process each batch of records
- global void finish(Database.BatchableContext bc){
- // execute any post-processing operations }
Key points about batch Apex
1.To use batch Apex, write an Apex class that implements Database.Batchable interface and make your class global
2.Implement the following 3 methods
- start
- execute
- finish
3.The default batch size is 200
Monitoring Batch Apex
To monitor or stop the execution of the batch Apex job, from Setup, enter Apex Jobs in the Quick Find box, then select Apex Jobs.
Batch class in salesforce
Here is example of Batch class in salesforce
- global class BatchApexExample implements Database.Batchable<sObject> {
- global Database.QueryLocator start(Database.BatchableContext BC) {
- // collect the batches of records or objects to be passed to execute
- String query = ‘SELECT Id, Name FROM Account’;
- return Database.getQueryLocator(query);
- global void execute(Database.BatchableContext BC, List<Account> accList) {
- // process each batch of records default size is 200
- for(Account acc : accList) {
- // Update the Account Name
- acc.Name = acc.Name + ‘sfdcpoint’;
- try {
- // Update the Account Record
- update accList;
- } catch(Exception e) {
- System.debug(e);
- global void finish(Database.BatchableContext BC) {
- // execute any post-processing operations like sending email
Invoking a Batch Class
To invoke a batch class, simply instantiate it and then call Database.executeBatch with the instance:
- MyBatchClass myBatchObject = new MyBatchClass();
- Id batchId = Database.executeBatch(myBatchObject);
You can also optionally pass a second scope parameter to specify the number of records that should be passed into the execute method for each batch. Pro tip: you might want to limit this batch size if you are running into governor limits.
- Id batchId = Database.executeBatch(myBatchObject, 100);
Each batch Apex invocation creates an AsyncApexJob record so that you can track the job’s progress. You can view the progress via SOQL or manage your job in the Apex Job Queue. We’ll talk about the Job Queue shortly.
- AsyncApexJob job = [SELECT Id, Status, JobItemsProcessed, TotalJobItems, NumberOfErrors FROM AsyncApexJob WHERE ID = :batchId ];
Scheduling Batch Apex
Can also use the Schedulable interface with batch Apex classes. The following example implements the Schedulable interface for a batch Apex class called batchable:
- global class scheduledBatchable implements Schedulable {
- global void execute(SchedulableContext sc) {
- BatchApexExample b = new BatchApexExample();
- Database.executeBatch(b);
Test class for batch Apex
- @isTest
- private class BatchApexExampleTest {
- static testmethod void test() {
- // Create test accounts to be updated by batch
- Account[] accList = new List();
- for (Integer i=0;i<400;i++) {
- Account ac = new Account(Name = ‘Account ‘ + i);
- accList.add(ac);
- insert accList;
- Test.startTest();
- BatchApexExample b = new BatchApexExample();
- Database.executeBatch(b);
- Test.stopTest();
- // Verify accounts updated
- Account[] accUpdatedList = [SELECT Id, Name FROM Account];
- System.assert(accUpdatedList[0].Name.Contains(‘sfdcpoint’));
Best Practices for batch Apex
As with future methods, there are a few things you want to keep in mind when using Batch Apex. To ensure fast execution of batch jobs, minimize Web service callout times and tune queries used in your batch Apex code. The longer the batch job executes, the more likely other queued jobs are delayed when many jobs are in the queue. Best practices include:
- Only use Batch Apex if you have more than one batch of records. If you don’t have enough records to run more than one batch, you are probably better off using Queueable Apex.
- Tune any SOQL query to gather the records to execute as quickly as possible.
- Minimize the number of asynchronous requests created to minimize the chance of delays.
- Use extreme care if you are planning to invoke a batch job from a trigger. You must be able to guarantee that the trigger won’t add more batch jobs than the limit.
In this section, you will understand about Batch Apex in Salesforce in detail. Consider a situation wherein you have to process large amounts of data on a daily basis and have to delete some unused data. It would be difficult to manually do so. This is where batching with Apex Salesforce comes to your rescue.
Before going deep into the concept of Batch Apex, let’s see the topics covered in this section:
- Advantages of Batch Apex in Salesforce
- Why use Batch Apex in Salesforce instead of the normal Apex?
- Batch Class in Salesforce
- Creating Apex Batch Class in Salesforce with an Example
Batch Apex in Salesforce is specially designed for a large number of records, i.e., here, data would be divided into small batches of records, and then it would be evaluated. In other words, Batch class in Salesforce is specifically designed to process bulk data or records together and have a greater governor limit than the synchronous code.
Advantages of Batch Apex in Salesforce
- Whenever a transaction is executed, Batch Apex ensures that the code stays within the governor limit.
- Until a batch is not successfully executed, Batch Apex won’t execute the following batches.
- A large set of records can be processed together on a regular basis using Batch Apex classes.
- The interface can be scheduled to run batches at different time periods.
- Asynchronous operations can be implemented by Batch Apex classes.
- Batch jobs are invoked programmatically during the runtime and can be operated on any size of records, with a maximum of 200 records per batch. You can break down a larger record data into 200 records per batch to execute it better.
Why use Batch Apex in Salesforce instead of the normal Apex?
There are various reasons why Batch Apex is better than normal Apex.
- SOQL queries: Normal Apex uses 100 records per cycle to execute SOQL queries. Whereas, Batch Apex does the same in 200 records per cycle.
- Retrieval of SOQL queries: Normal Apex can retrieve 50,000 SOQL queries but, in Batch Apex, 50,000,000 SOQL queries can be retrieved.
- Heap size: Normal Apex has a heap size of 6 MB; whereas, Batch Apex has a heap size of 12 MB.
- Errors: When executing bulk records, Normal Apex classes are more vulnerable to encountering errors as compared to Batch Apex.
Batch Class in Salesforce
When using a Batch class in Salesforce, the batchable interface needs to be implemented first. It has the following three methods:
- Start: This method is called at the starting of a batch job to collect the data on which the batch job will be operating. It breaks the data or record into batches. In some cases, the ‘QueryLocator’ method is used to operate with the simple SOQL query to generate the scope of objects inside a batch job.
- Syntax: global void execute(Database.BatchableContext BC, list<sobject<) {}
- Execute: This method executes after the Start method, and it does the actual processing for each batch, separately.
- Syntax: global void execute(Database.BatchableContext BC, list<sobject<) {}
- Finish: This method will be called at last. Since this method is called in the end, it is responsible to perform post-processing operations such as sending an email. When this process is called all batches are already executed.
- Syntax: global void finish(Database.BatchableContext BC) {}
Creating an Apex Batch Class in Salesforce with an Example
Step 1: Search for Apex Salesforce Classes in Setup

Step 2: Once you open Salesforce Apex Classes, click on New to create a new Apex Class
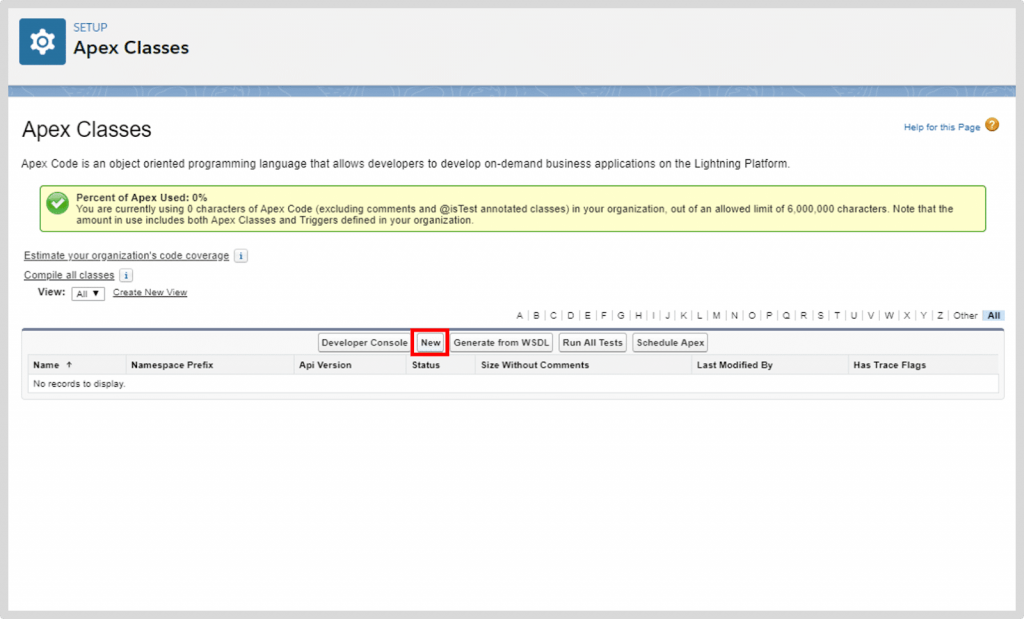
Step 3: Below is the screen on which you’ll have to write your code

The following is the program that updates the account name in the account object with the keyword ‘Updated’ after the account name.

- // Batch Job for Processing the Records
- global class batchAccountUpdate implements Database.Batchable<sObject>
- // Start Method
- global Database.QueryLocator start(Database.BatchableContext BC)
- String query = ‘SELECT Id,Name FROM Account’;
- return Database.getQueryLocator(query);
- // Execute method
- global void execute(Database.BatchableContext BC, List<Account>
- for(Account a : scope)
- {a.Name = a.Name + ‘Updated’;
- update scope;
- // Finish Method
- global void finish(Database.BatchableContext BC)
Step 4: After writing the code, you’ll have to go to Developer Console and click on Debug and then on Open Execute Anonymous Window. You’ll see the following screen:

- Enter the following code in the box and click on Execute
- batchAccountUpdate b = new batchAccountUpdate();
- database.executeBatch(b);
- Each transaction is executed with a set of defined governor limits, batch Apex concept makes sure that code should stay within governor limits only.
- In case, one batch is not executed successfully then it will not roll back transactions of other batches.
- If you wanted to process a large set of records together on regular basis then you should opt for the batch apex classes.
- When you want an operation to be asynchronous then implement the batch apex class. The interface can be implemented by developers only. The batch jobs are invoked programmatically during runtime. It can be operated on any size of records, but you can add a maximum of 200 records per batch. If the size of the recordset is larger then 200 then break it down into small manageable chunks.
- Most importantly, the interface can be scheduled to run batches at different time periods.
- There is a maximum of five active or queued batch jobs are allowed for APEX.
- At a particular time period, one user can open up to 50 query cursors. If the user attempts to open a new one the oldest 50 cursors will be released. Keep in mind that this limit is different for start method in the Batch APEX class that could have a maximum of five query cursors open at a particular time.
- Other than the start method, the rest of the methods have limit up to 50 cursors. This limit is tracked differently for multiple features in Force.com. For example, for a particular method, you could have 50 batch cursors, 50 Apex query cursors, and 50 VisualForce cursors open at the same time together.
- For a database in the batch APEX, a maximum of 50 million records can be returned at a particular time. Once all 50 million records are returned, the batch job will be terminated automatically and it is marked as the “Failure” in the end.
- The default size for a record set in batch APEX is 200. The governor limits should always be redefined for each set of records as per the requirement.
- When we are talking about the callouts then start, execute, and the finish methods are limited to ten callouts per method execution.
- There is a limit set for hours as well. For example, you can execute a maximum number of 250,000 batch execution in 24 hours.
- At one time, only one start method will run for an organization. The batch jobs that are not started yet will stay in queue until they are not started. Keep in mind that this limit will not set any batch job to failure or it will not stop the execution of any batch APEX jobs but running in parallel if there are more than one job scheduled in the batch.
- Use batch APEX class only when you have more than one batch of records to execute. If you don’t have enough record sets then you may probably turn off the batch APEX idea and continue with normal context.
- Tune SOQL queries together to gather records to be executed as quickly as possible.
- Try to minimize the total number of asynchronous requests to minimize the chance of delays.
- Add extra care when planning to invoke a batch job from a trigger. Here, you have to keep in mind that the trigger won’t be able to add more batch jobs than the specified governor limits.
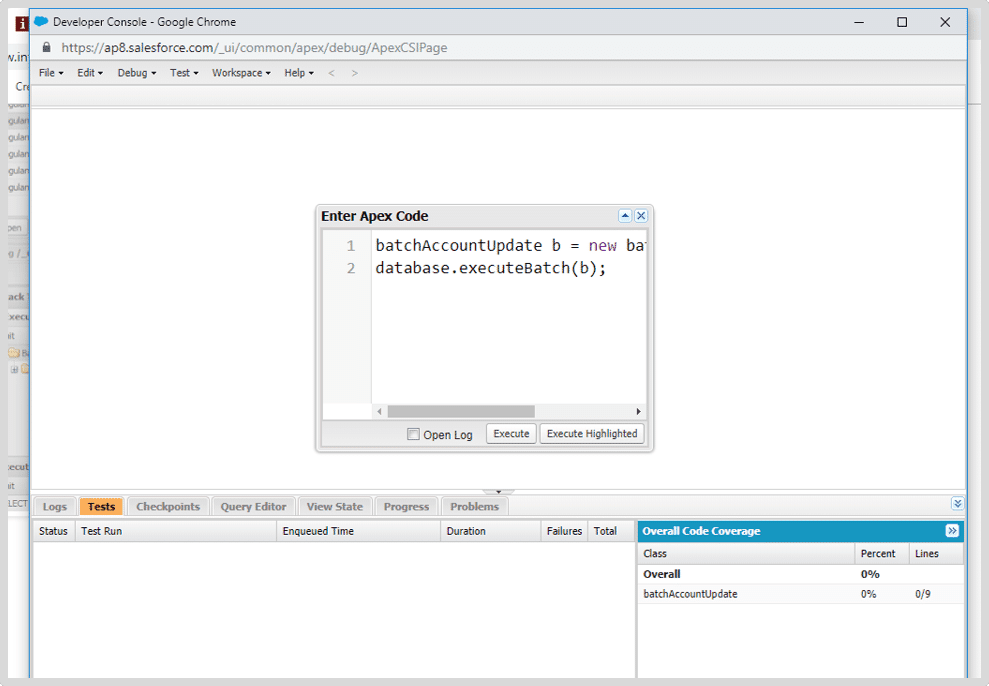
Why batch apex is better than normal apex classes?
Area | Normal Apex | Batch Apex |
---|---|---|
SOQL Queries | 100 records per cycle | 200 records per cycle |
Records retrieval by SOQL Queries | 50,000 | 50,000,000 |
Heap Size | 6MB | 12MB |
When to use Batch Apex?
Batch Apex is an essential requirement when you wanted to execute bulk records, if you are using regular apex classes then chances of errors are higher and it may hit the governor limits too. A maximum of 200 records can be passed to every batch here. The concept has plenty of awesome advantages that you should check before moving ahead –
Governor Limits Defined for Batch Apex
Before you write batch apex code, you should focus on these governor limits for effective dealing with classes.
How to write a Batch APEX Class?
To write a batch APEX class, you should first implement the “Database.Batchable” interface in the Salesforce and including the execution of these three methods.
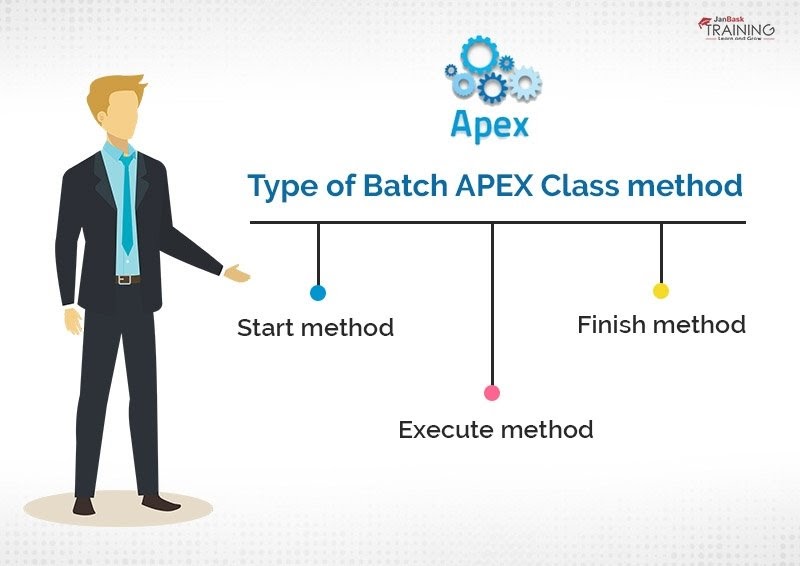
1). Start method
This method is used to collect objects or records that need to be passed to an interface method for processing. This method is called at the beginning when Batch apex starts to execute. In most of the cases, the “QueryLocator” method is used to play with the simple SOQL query and to generate the scope of objects within a batch job. In case, you wanted to do something crazy then call the API records before they are passed for the execution. Further, if you wanted to set the governor limits for a total number of records retrieved by the SOQL queries then you can query up to 50 million records together. With the help of an iterable object, the governor limit for a total number of records is retrieved by SOQL queries through enforcement.
2). Execute Method
This method will perform the actual processing for each batch or chunk where data needs to be passed with the help of a method. The default size defined for each batch is 200 records maximum. If the size of recordset is larger, break it down into manageable pieces first before you execute them. If the size is not defined well then records will not be executed in the same order as they were received by the start method.
3). Finish method
This method is responsible to perform post-processing operations like email sending or more once all batches are executed successfully. Here is the basic syntax of a batch APEX class how are they written in the Salesforce.
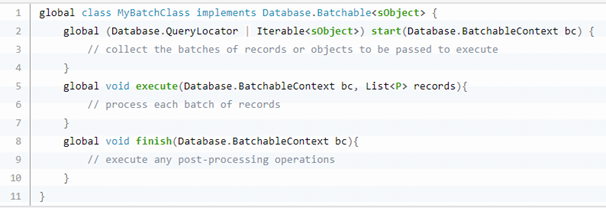
How to use state in batch apex class?
A batch apex class is usually stateless and execution of each job in the batch is considered a discrete transaction. For example, if some batch class contains more than 1000 records then based on the default size concept total five transactions will be performed here with a recordset of 200 each. Here you need to specify the Database. The stateful method in the class definition to maintain the state across all transactions. With the help of this method, the member variables could always retain their values between multiple transactions. You must be thinking why it is necessary but maintaining state is beneficial to summarize records as they are processed. For example, when we are updating contact records in our batch then you wanted to keep track of the total records affected so that the same can be included for the notification email list. Now you know how to write a batch class with the help of three methods and defining state for the database. Don’t forget to practice a few examples to get a better idea of the concept. Keep in mind that APEX development and testing go hand to hand. Every time you add some records, call the batch APEX class and check if contacts are updated properly or not as per the specified requirements.
Best Practices:
As discussed earlier, there are always certain things you should keep in mind when using the Batch Apex class. To make sure that batch jobs are executing faster, you should minimize the callout times for web services and tune the queries used with your batch APEX code. The longer the batch size would be, the more likely other job queues will be delayed when too many jobs are added together. Here are the best practices to follow by Salesforce Developers:
Conclusion
With this discussion, you have covered almost every concept related to batch apex class. Now, you can use it successfully in your next project and don’t forget to define governor limits well otherwise it may be annoying at the end. Today, batch apex class usage is growing tremendously and Salesforce developers are also excited about its benefits.
Are you looking training with Right Jobs?
Contact Us- Salesforce Architecture Tutorial
- What Does The Future Hold For Salesforce?
- Salesforce Developer Tutorial
Related Articles
Popular Courses
- VM Ware Training
11025 Learners
- Microsoft Dynamics Training
12022 Learners
- Siebel Training
11141 Learners
- What is Dimension Reduction? | Know the techniques
- Difference between Data Lake vs Data Warehouse: A Complete Guide For Beginners with Best Practices
- What is Dimension Reduction? | Know the techniques
- What does the Yield keyword do and How to use Yield in python ? [ OverView ]
- Agile Sprint Planning | Everything You Need to Know