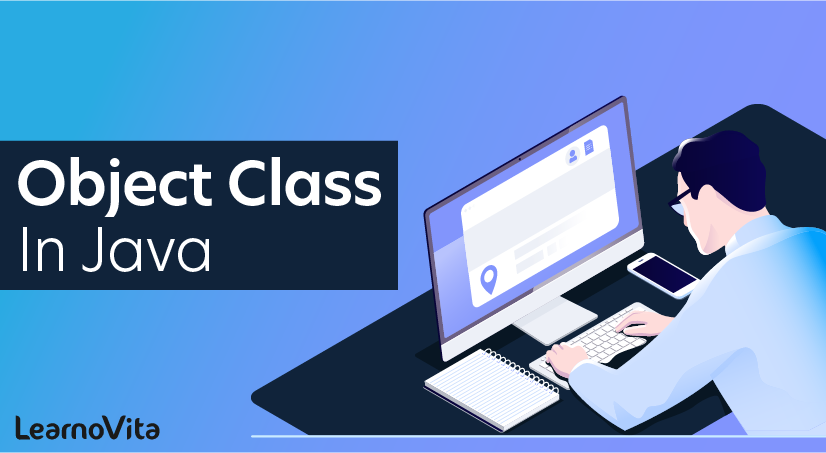
Object Class in Java
Last updated on 21st Sep 2020, Artciles, Blog
- Object is an instance of a class while class is a blueprint of an object. An object represents the class and consists of properties and behavior.
- Properties refer to the fields declared within class and behavior represents to the methods available in the class.
- In the real world, we can understand an object as a cell phone that has its properties like: name, cost, color etc and behavior like calling, chatting etc.
- So we can say that an object is a real world entity.
- Some real world objects are: ball, fan, car etc.

Subscribe For Free Demo
Error: Contact form not found.
Java Object Syntax
- className variable_name = new className();
- Here, className is the name of a class that can be anything like: Student that we declared in the above example.
- variable_name is the name of the reference variable that is used to hold the reference of the created object.
- The new is a keyword which is used to allocate memory for the object.
Example
Lets see an example to create an object of class Student that we created in the above class section.
Although there are many other ways by which we can create objects of the class. We have covered this section in detail in separate topics.
Example: Object creation
- Student std = new Student();
- Here, std is an object that represents the class Student during runtime.
- The new keyword creates an actual physical copy of the object and assigns it to the std variable. It will have physical existence and get memory in the heap area.
- The new operator dynamically allocates memory for an object.

In this image, we can get an idea how an object refers to the memory area.
Example: Creating a Class and its object
-
public class Student
{
String name;
int rollno;
int age;
void info()
{
System.out.println(“Name: “+name);
System.out.println(“Roll Number: “+rollno);
System.out.println(“Age: “+age);
}
public static void main(String[] args) {
Student student = new Student();// Accessing and property value
student.name = “Ramesh”;
student.rollno = 253;
student.age = 25;
student.info(); // Calling method
}}
Output
Name: Ramesh
Roll Number: 253
Age: 25
In this example, we created a class Student and an object. Here you may be surprised to see the main() method but don’t worry it is just an entry point of the program by which JVM starts execution.
Here, we used the main method to create objects of the Student class and access its fields and methods.
Example: Class fields
public class Student{
String name;
int rollno;
int age;
void info()
{
System.out.println(“Name: “+name);
System.out.println(“Roll Number: “+rollno);
System.out.println(“Age: “+age);
}
public static void main(String[] args) {
Student student = new Student();// Calling method
student.info();
}}
Output
Name: null
Roll Number: 0
Age: 0
In case, if we don’t initialized values of class fields then they are initialized with their default values.
Number of ways to create an object in Java
- Using new keyword: It is the simplest, common and regular way to create an object in Java. By using this object creation method we can call any default or parameterized constructor. This object creation way is required when you are passing values to the parameterized constructor at the time of an object creation.
Example:
Student stud1 = new Student(11, “Rajveer”);
In the above example stud1 is a reference variable that refer to the created object, new keyword is responsible to allocate memory to the object. In the statement a 2 parameterized constructor will get called.
2. Using newInstance() method of Class class: The Class class has a newInstance() method to create an object of a class. This newInstance() method calls the default or no-argument constructor to create the Java object.
We can create an Java object by newInstance() method like below
Example:
Student stud = (Student)Class.forName(“Student”).newInstance();
Or:
Student stud = Student.class.newInstance();
3. Using newInstance() method of Constructor class: The java.lang.reflect. The Constructor class has a newInstance() method to create an java object. In following way we can use newInstance() method of Constructor class to create an object.
- Constructor
constructor= Student.class.getConstructor(); - Student stud = constructor.newInstance();
The above two ways are reflective ways to create an java object using newInstance() method. The newInstance() method of the Class class internally uses the newInstance() method of the Constructor class. The newInstance() method of Constructor class is preferred and used by different frameworks like Spring, Hibernate, Struts, etc.
4. Using clone() method: The Object class has a protected clone() method, if we call clone() on any object, the JVM internally creates a new java object and copies all content of the existing object into new one. This way of creating an object using the clone() method does not invoke any constructor.
To use clone() method on an object we need to implement Cloneable interface and override the clone() method into that class. If we call clone() method on a object and that class is not implementing the Cloneable interface then we will get CloneNotSupportedException.
Student stud = (Student) oldstud.clone();
5. Using deserialization: Whenever we serialize and deserialize an object, the JVM creates a separate object. In deserialization, the JVM doesn’t use any constructor to create the object.
To serialize and deserialize an object we need to implement the Serializable interface in our class.
- ObjectInputStream in = new ObjectInputStream(new FileInputStream(“sample.txt”));
- Student stud = (Student) in.readObject();
Are you looking training with Right Jobs?
Contact Us- The Most Popular Java Applications Used World-wide
- Resources To Help You Learn Java Programming
- Top Skills that Boosts Java Developer Salaries
- Java Interview Questions and Answers
- JAVA Tutorial
Related Articles
Popular Courses
- Python Online Training
11025 Learners
- C And C Plus Plus Online Training
12022 Learners
- C Sharp Online Training
11141 Learners
- What is Dimension Reduction? | Know the techniques
- Difference between Data Lake vs Data Warehouse: A Complete Guide For Beginners with Best Practices
- What is Dimension Reduction? | Know the techniques
- What does the Yield keyword do and How to use Yield in python ? [ OverView ]
- Agile Sprint Planning | Everything You Need to Know